Consider the case: There is a base class BASE, and a derived class DERIVED. Both have a member function getInfo(). It's a array of pointer to BASE object. Because object of DERIVED "is a" BASE object also, so it can be pointed by the array.
When we call the getInfo() function of DERIVED object pointed by a pointer to BASE, which function will be called? BASE::getInfo()? or DERIVED::getInfo()? - The answer is BASE::getInfo()!
It's also happen on the destructor, when we delete a DERIVED object pointed by a pointer to BASE, the destructor of BASE will be called.
It's called static binding.
#include <iostream>
using namespace std;
class BASE{
public:
BASE(int x, int y, int z);
~BASE();
void getInfo();
int a;
protected:
int b;
string Im;
private:
int c;
};
BASE::BASE(int x, int y, int z)
:a(x), b(y), c(z){
Im = "Base Class";
}
BASE::~BASE(){
cout << "BASE's destructor! " << Im << endl;
}
void BASE::getInfo(){
cout << "BASE::getInfo()" << " : " << Im << endl;
}
class DERIVED:public BASE{
public:
DERIVED(int x, int y, int z, int w);
~DERIVED();
void getInfo();
private:
int d;
};
DERIVED::DERIVED(int x, int y, int z, int w)
:BASE(x, y, z), d(w){
Im = "Derived Class";
}
DERIVED::~DERIVED(){
cout << "DERIVED's destructor! " << Im << endl;
}
void DERIVED::getInfo(){
cout << "DERIVED::getInfo()" << " : " << Im << endl;
}
int main()
{
BASE* base[2];
base[0] = new BASE(1, 2, 3);
base[1] = new DERIVED(4, 5, 6, 7);
base[0]->getInfo();
base[1]->getInfo();
cout << endl;
cout << "delete base[0]" << endl;
delete base[0];
cout << endl;
cout << "delete base[1]" << endl;
delete base[1];
return 0;
}
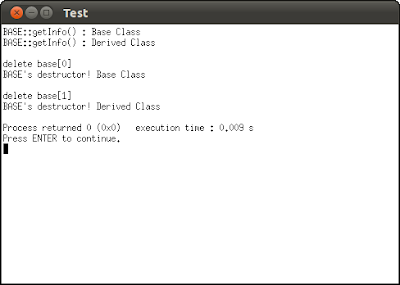
To solve this problem, we can define both the getInfo() and destructor as virtual, to specify dynamic binding on the functions.
#include <iostream>
using namespace std;
class BASE{
public:
BASE(int x, int y, int z);
virtual ~BASE();
virtual void getInfo();
int a;
protected:
int b;
string Im;
private:
int c;
};
BASE::BASE(int x, int y, int z)
:a(x), b(y), c(z){
Im = "Base Class";
}
BASE::~BASE(){
cout << "BASE's destructor! " << Im << endl;
}
void BASE::getInfo(){
cout << "BASE::getInfo()" << " : " << Im << endl;
}
class DERIVED:public BASE{
public:
DERIVED(int x, int y, int z, int w);
virtual ~DERIVED();
virtual void getInfo();
private:
int d;
};
DERIVED::DERIVED(int x, int y, int z, int w)
:BASE(x, y, z), d(w){
Im = "Derived Class";
}
DERIVED::~DERIVED(){
cout << "DERIVED's destructor! " << Im << endl;
}
void DERIVED::getInfo(){
cout << "DERIVED::getInfo()" << " : " << Im << endl;
}
int main()
{
BASE* base[2];
base[0] = new BASE(1, 2, 3);
base[1] = new DERIVED(4, 5, 6, 7);
base[0]->getInfo();
base[1]->getInfo();
cout << endl;
cout << "delete base[0]" << endl;
delete base[0];
cout << endl;
cout << "delete base[1]" << endl;
delete base[1];
return 0;
}